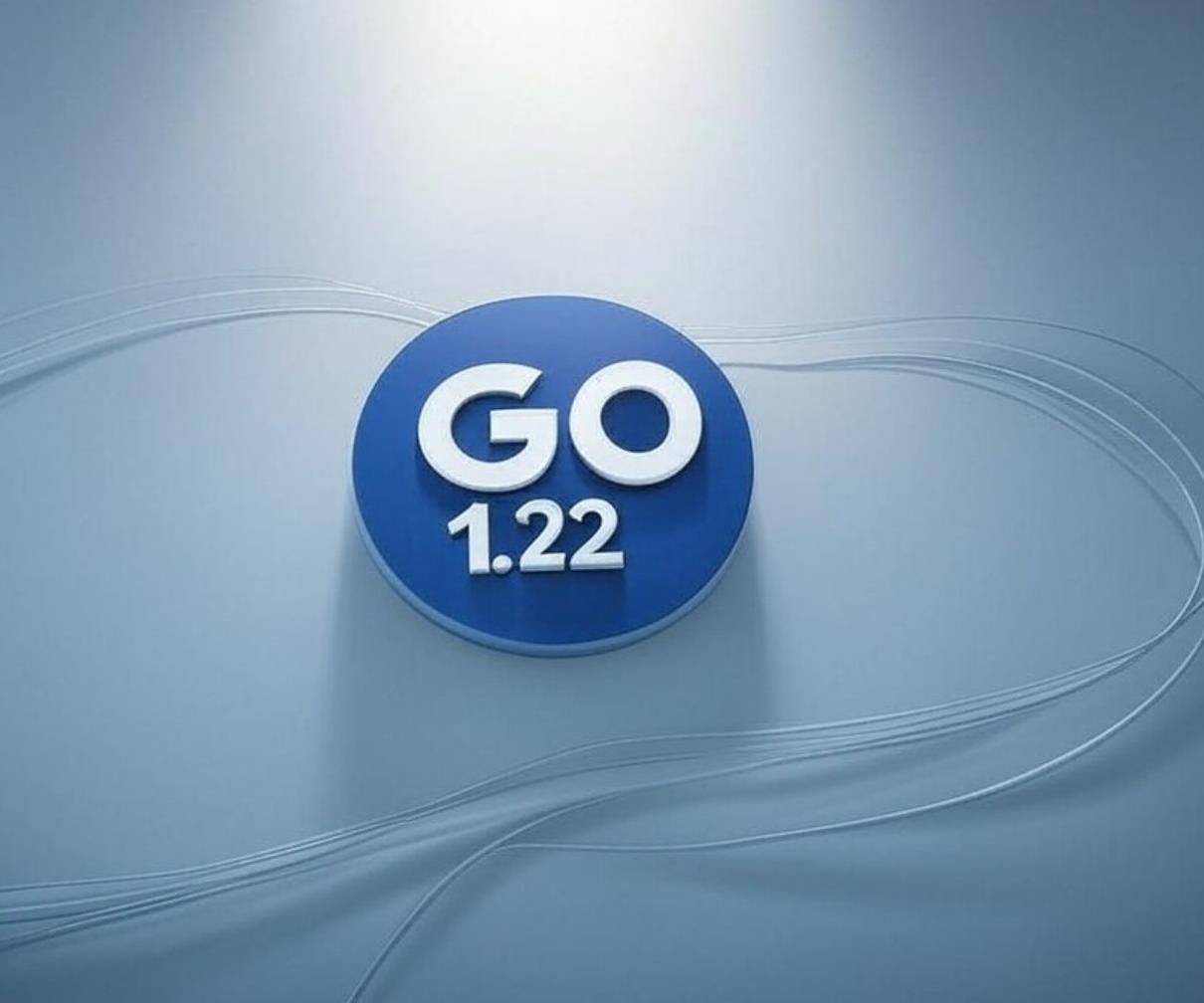
Notes
Go 1.22: A New Era of Routing Simplicity
Introduction
Go 1.22 introduces a game-changing improvement to web routing with a more intuitive and powerful ServeMux
syntax. This article explores the new routing capabilities and demonstrates how they simplify web application development.
Old vs New: Routing Syntax Comparison
Traditional Routing (Pre-1.22)
func main() {
http.HandleFunc("/users", func(w http.ResponseWriter, r *http.Request) {
// Complex manual parsing of paths and methods
if r.Method != http.MethodGet {
http.Error(w, "Method not allowed", http.StatusMethodNotAllowed)
return
}
})
}
New Routing in Go 1.22
func main() {
mux := http.NewServeMux()
mux.HandleFunc("GET /users/{id}", func(w http.ResponseWriter, r *http.Request) {
// Automatic method and path parameter handling
id := r.PathValue("id")
// Clean, straightforward code
})
}
Demonstration Project: Note Management API
Let's create a simple note management application that showcases the new routing capabilities.
Project Structure
notes-app/
├── compose.yml
├── Dockerfile
└── main.go
compose.yml:
services:
app:
build: .
ports:
- "8080:8080"
Dockerfile:
FROM golang:1.22-alpine
WORKDIR /app
COPY go.mod main.go ./
RUN go build -o notes-app
EXPOSE 8080
CMD ["./notes-app"]
main.go:
package main
import (
"encoding/json"
"net/http"
)
type Note struct {
ID string `json:"id"`
Content string `json:"content"`
}
var notes = map[string]Note{
"1": {ID: "1", Content: "First note"},
}
func main() {
mux := http.NewServeMux()
mux.HandleFunc("GET /notes/{id}", func(w http.ResponseWriter, r *http.Request) {
id := r.PathValue("id")
note, ok := notes[id]
if !ok {
http.NotFound(w, r)
return
}
json.NewEncoder(w).Encode(note)
})
mux.HandleFunc("POST /notes", func(w http.ResponseWriter, r *http.Request) {
var note Note
json.NewDecoder(r.Body).Decode(¬e)
note.ID = "2"
notes[note.ID] = note
w.WriteHeader(http.StatusCreated)
})
http.ListenAndServe(":8080", mux)
}
Running the Project
To run the project:
```
go mod init my.go-routing
docker compose up --build
http://localhost:8080/notes/1
```
Key Advantages of the New Routing Syntax
- Method-Specific Routing: Directly specify HTTP methods in route definitions
- Path Parameters: Easily extract path values with
r.PathValue()
- Simplified Matching: More intuitive route pattern matching
- Built-in Support: No need for third-party routing libraries
Conclusion
Go 1.22's new routing syntax represents a significant improvement in web application development. It simplifies route handling, reduces boilerplate code, and provides a more intuitive approach to defining web service endpoints.
By embracing this new syntax, developers can write more readable and maintainable web services with less complexity.
Recommendations
- Upgrade to Go 1.22 to leverage these routing improvements
- Start refactoring existing routing code to use the new
ServeMux
- Explore more advanced routing patterns and combinations
Happy coding! 🚀
Афоризм дня:
Надо уметь переносить то, чего нельзя избежать. (506)
By den
On December 12, 2024