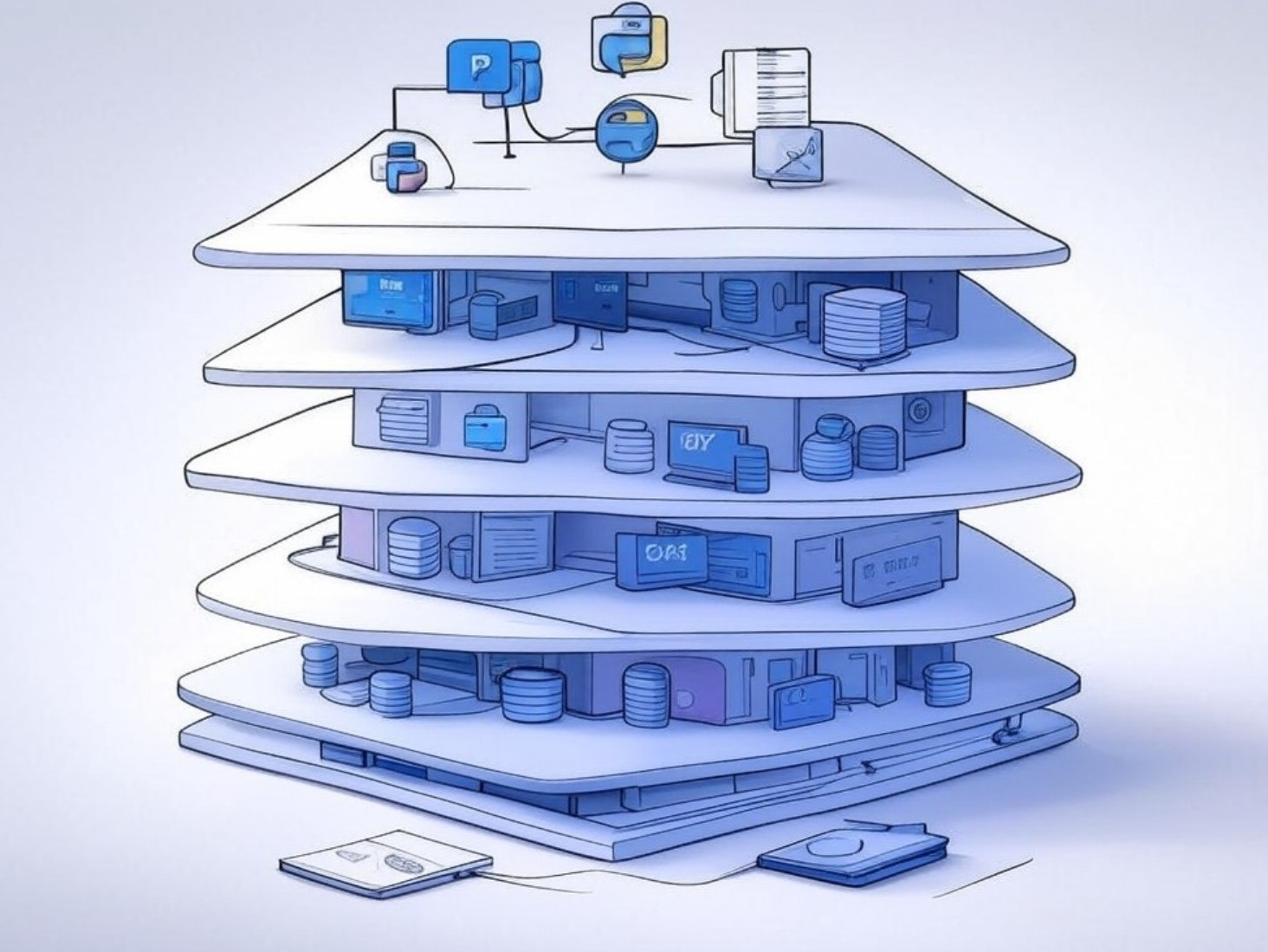
Notes
Structuring Enterprise Python Projects 2025: Flask/FastAPI with Clean Architecture Principles
Here's a combined structure, blending Clean Architecture principles with Flask/FastAPI recommendations, optimized for enterprise-level Python web applications:
Project Structure:
project/
├── app/
│ ├── __init__.py # Application factory (if using Flask) or app creation logic
│ ├── main.py # Main entry point (FastAPI/Flask app instance)
│ ├── api/
│ │ ├── __init__.py # API versioning support
│ │ ├── v1/ # API v1
│ │ │ ├── endpoints/ # Route handlers for v1
│ │ │ │ ├── __init__.py
│ │ │ │ ├── user.py # Example: User endpoints
│ │ │ │ └── product.py # Example: Product endpoints
│ │ │ ├── schemas/ # Pydantic models for validation
│ │ │ │ ├── __init__.py
│ │ │ │ ├── user.py
│ │ │ │ └── product.py
│ │ └── v2/ # Future API versions
│ │ ├── endpoints/
│ │ ├── schemas/
│ │ └── ...
│ ├── core/
│ │ ├── config.py # Configuration management (e.g., reading env vars)
│ │ ├── security.py # Authentication and authorization logic
│ │ └── exceptions.py # Custom exception handlers
│ ├── db/
│ │ ├── __init__.py # Database initialization
│ │ ├── base.py # Base class for ORM models (e.g., SQLAlchemy)
│ │ ├── models/
│ │ │ ├── __init__.py
│ │ │ ├── user.py
│ │ │ └── product.py
│ │ └── migrations/ # Database migration scripts
│ │ ├── env.py
│ │ └── versions/
│ ├── domain/ # Core business logic (agnostic to frameworks)
│ │ ├── entities/ # Core entities
│ │ │ ├── __init__.py
│ │ │ ├── user.py # User entity (pure business logic)
│ │ │ └── product.py # Product entity
│ │ ├── repositories/ # Repository interfaces
│ │ │ ├── __init__.py
│ │ │ ├── user.py
│ │ │ └── product.py
│ │ ├── services/ # Business use cases
│ │ │ ├── __init__.py
│ │ │ ├── user_service.py
│ │ │ └── product_service.py
│ │ └── exceptions.py # Domain-specific exceptions
│ ├── infrastructure/ # Framework and implementation details
│ │ ├── repositories/ # Concrete repository implementations
│ │ │ ├── __init__.py
│ │ │ ├── user_repo.py # SQLAlchemy implementation for user repository
│ │ │ └── product_repo.py # SQLAlchemy implementation for product repository
│ │ ├── email/ # Email sending logic
│ │ │ ├── __init__.py
│ │ │ └── sendgrid.py # Example: SendGrid integration
│ │ ├── storage/ # File storage logic (e.g., S3)
│ │ ├── tasks/ # Background tasks (e.g., Celery)
│ │ └── third_party/ # External API integrations
│ └── utils/ # Shared utility functions
│ ├── __init__.py
│ ├── logger.py # Logging configuration
│ └── helpers.py # General helper functions
├── tests/
│ ├── __init__.py
│ ├── unit/ # Unit tests
│ │ ├── test_user.py
│ │ └── test_product.py
│ ├── integration/ # Integration tests
│ │ ├── test_api_v1.py
│ │ └── test_db.py
│ ├── e2e/ # End-to-end tests
│ │ └── test_full_flow.py
│ └── conftest.py # Pytest fixtures
├── config/ # Environment-specific configurations
│ ├── base.py # Shared settings
│ ├── dev.py # Development-specific settings
│ └── prod.py # Production-specific settings
├── scripts/ # One-off scripts and utilities
│ ├── migrate.py # Database migration runner
│ ├── seed_data.py # Script for seeding initial data
│ └── clean_logs.py # Example: Log cleanup script
├── docker/ # Docker-related files
│ ├── Dockerfile # Base Dockerfile
│ ├── docker-compose.yml # Docker Compose configuration
│ └── entrypoint.sh # Entrypoint script
├── docs/ # Documentation
│ ├── architecture.md # Architecture documentation
│ └── api_reference.md # API reference
├── .env # Environment variables (local development)
├── pyproject.toml # Dependency and build configuration (Poetry support)
├── README.md # Project overview and instructions
└── .gitignore # Ignored files
Key Features of This Structure:
-
Separation of Concerns:
- Domain Layer: Pure business logic.
- Infrastructure Layer: Implementation details (databases, email, storage, etc.).
- API Layer: Handles HTTP requests and responses.
-
Scalability:
- Modular structure ensures easy expansion of APIs, domain logic, and integrations.
-
Testing:
- Tests are organized into
unit
,integration
, ande2e
for clarity. - Fixtures in
conftest.py
support reusability.
- Tests are organized into
-
Configuration Management:
- Centralized
config/
directory with environment-specific settings (base.py
,dev.py
,prod.py
).
- Centralized
-
Modern Tools:
pyproject.toml
: Centralized configuration for dependencies and build.- Docker support for reproducible deployments.
-
Framework-Agnostic Design:
- The domain layer is isolated and can be reused or tested independently of Flask/FastAPI.
When to Use This Structure:
- Enterprise-grade projects requiring high scalability.
- Web applications with complex business logic.
- Teams that want clear boundaries between layers for testability and maintainability.
This structure combines the best of Clean Architecture and practical needs for modern Python web development.
Афоризм дня:
Надежда выздороветь – половина выздоровления. (511)
By den
On January 28, 2025