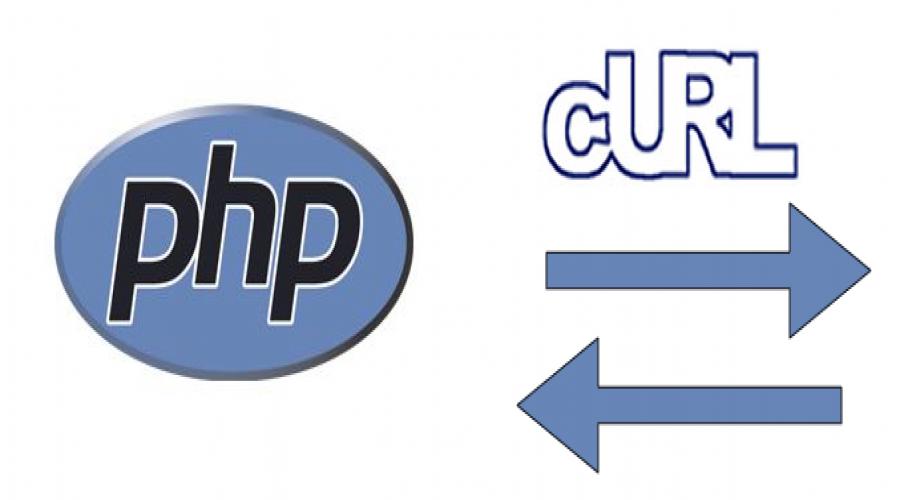
PHP
Класс для работы с CURL на PHP
Создадим универсальный класс для HTTP запросов GET/POST/PUT на основе CURL.
(пока еще не по PSR-7)
Сам класс:
/**
* Class Client
* @package App\Helpers
*/
class Client1
{
public function request($method, $url, $body = [])
{
$method = strtoupper($method);
//$headers = request()->headers->all();
try {
return $this->curl($method, $url, $body);
} catch (Throwable $e) {
return $e->getMessage();
}
}
private $connections = [];
/**
* @throws JsonException
*/
private function curl(
string $method,
string $url,
array $bodyFields = [],
array $headers = []
): Response {
$connectionKey = $url . $method
. json_encode($bodyFields, JSON_THROW_ON_ERROR)
. json_encode($headers, JSON_THROW_ON_ERROR);
if (empty($this->connections[$connectionKey])) {
$curl = curl_init();
curl_setopt_array(
$curl,
[
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => $method,
CURLOPT_HTTPHEADER => $this->prepareHeaders($headers),
CURLOPT_POSTFIELDS => is_array($bodyFields)
? json_encode($bodyFields, JSON_THROW_ON_ERROR | JSON_UNESCAPED_UNICODE)
: $bodyFields,
]
);
$this->connections[$connectionKey] = $curl;
} else {
$curl = $this->connections[$connectionKey];
}
$responseBody = curl_exec($curl);
$statusCode = curl_getinfo($curl, CURLINFO_HTTP_CODE);
//curl_close($curl);
return new Response('', $responseBody, $statusCode);
}
private function prepareHeaders($headers): array
{
$flattened = [];
foreach ($headers as $key => $header) {
if (is_int($key)) {
$flattened[] = $header;
} else {
$flattened[] = $key . ': ' . $header;
}
}
return $flattened;//implode("\r\n", $flattened);
}
}
class Response
{
private $body;
private $headers;
private $statusCode;
public function __construct($headers, $body, $statusCode)
{
$this->headers = $headers;
$this->body = $body;
$this->statusCode = $statusCode;
}
public function getBody()
{
return $this->body;
}
public function getHeaders()
{
return $this->headers;
}
public function getStatusCode()
{
return $this->statusCode;
}
}
Пример использования:
$proxy = Settings::get($name, 'service_proxy');
if ($proxy) {
$url = $proxy;
$msg = Proxy::request($url, 'POST', json_decode($request->getContent(), 1));
return response($msg)->header('Content-Type', 'application/json');
}
Афоризм дня:
Комедия имеет намерение отображать людей худших, а трагедия – лучших, чем существующие. (647)
By Admin
On December 18, 2018